主要讲解
BeanFactory
实现特点
ApplicationContext
的常见实现和用法
- 内嵌容器、注册
DispatcherServlet
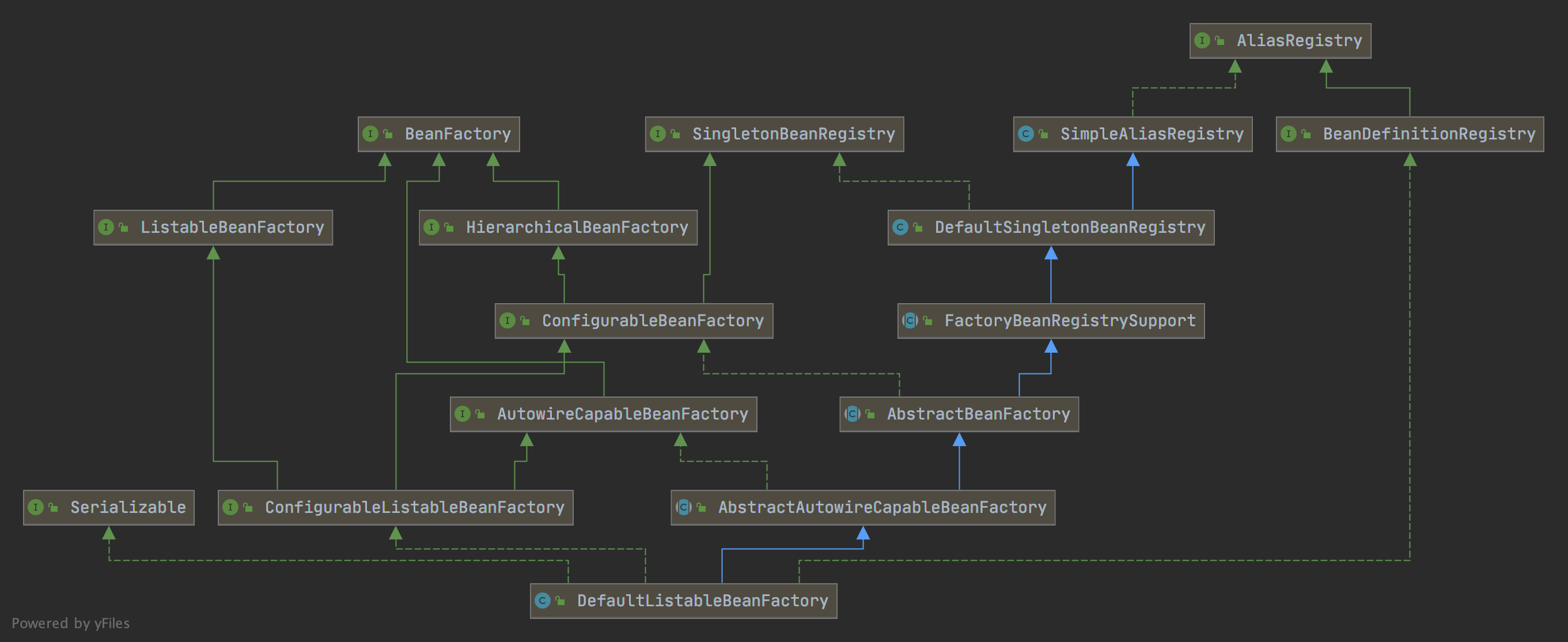
BeanFactory
一个重要的实现是 DefaultListableBeanFactory
。
BeanFactory 作用
- 不会主动调用
BeanFactory
后处理器
- 不会主动添加
Bean
后处理器
- 不会主动初始化单例
- 不会解析
beanFactory
,也不会解析 ${}
与 #{}
下面会自己创建一个 beanFactory 来模拟 spring boot 自加载整个过程。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114
| package com.lou.springboot;
import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.config.BeanFactoryPostProcessor; import org.springframework.beans.factory.config.BeanPostProcessor; import org.springframework.beans.factory.support.AbstractBeanDefinition; import org.springframework.beans.factory.support.BeanDefinitionBuilder; import org.springframework.beans.factory.support.DefaultListableBeanFactory; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.context.ConfigurableApplicationContext; import org.springframework.context.annotation.AnnotationConfigUtils; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration;
import java.io.IOException;
@SpringBootApplication public class Application {
public static void main(String[] args) throws NoSuchFieldException, IllegalAccessException, IOException { DefaultListableBeanFactory beanFactory = new DefaultListableBeanFactory();
System.out.println("==============初始化 config =================="); AbstractBeanDefinition beanDefinition = BeanDefinitionBuilder.genericBeanDefinition(Config.class).setScope("singleton").getBeanDefinition(); // beanFactory 里面有一个叫做 config 的 bean beanFactory.registerBeanDefinition("config", beanDefinition); for (String name : beanFactory.getBeanDefinitionNames()) { System.out.println(name); } System.out.println("===============添加后处理器=================");
AnnotationConfigUtils.registerAnnotationConfigProcessors(beanFactory); for (String name : beanFactory.getBeanDefinitionNames()) { System.out.println(name); } System.out.println("==============添加 beanFactory 后处理器==================");
beanFactory.getBeansOfType(BeanFactoryPostProcessor.class).values().stream().forEach( beanFactoryPostProcessor -> { beanFactoryPostProcessor.postProcessBeanFactory(beanFactory); } ); for (String name : beanFactory.getBeanDefinitionNames()) { System.out.println(name); } System.out.println("=============添加 bean 后处理器 ===================");
beanFactory.getBeansOfType(BeanPostProcessor.class).values().forEach( beanFactory::addBeanPostProcessor );
for (String name : beanFactory.getBeanDefinitionNames()) { System.out.println(name); }
System.out.println("================查看输出================"); for (String name : beanFactory.getBeanDefinitionNames()) { System.out.println(name); }
System.out.println(beanFactory.getBean(Bean1.class).getBean2());
}
@Configuration static class Config { @Bean public Bean1 bean1() { return new Bean1(); }
@Bean public Bean2 bean2() { return new Bean2(); } }
static class Bean1 { private static final Logger log = LoggerFactory.getLogger(Bean1.class);
public Bean1() { log.debug("构造 Bean1"); }
@Autowired private Bean2 bean2;
public Bean2 getBean2() { return bean2; } }
static class Bean2 { private static final Logger log = LoggerFactory.getLogger(Bean2.class);
public Bean2() { log.debug("构造 Bean2"); } }
}
|
输出
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| ==============初始化 config ================== config ===============添加后处理器================= config org.springframework.context.annotation.internalConfigurationAnnotationProcessor org.springframework.context.annotation.internalAutowiredAnnotationProcessor org.springframework.context.annotation.internalCommonAnnotationProcessor org.springframework.context.event.internalEventListenerProcessor org.springframework.context.event.internalEventListenerFactory ==============添加 beanFactory 后处理器================== 20:18:25.235 [main] DEBUG org.springframework.beans.factory.support.DefaultListableBeanFactory - Creating shared instance of singleton bean 'org.springframework.context.annotation.internalConfigurationAnnotationProcessor' 20:18:25.251 [main] DEBUG org.springframework.beans.factory.support.DefaultListableBeanFactory - Creating shared instance of singleton bean 'org.springframework.context.event.internalEventListenerProcessor' 20:18:25.367 [main] DEBUG org.springframework.beans.factory.support.DefaultListableBeanFactory - Creating shared instance of singleton bean 'org.springframework.context.event.internalEventListenerFactory' config org.springframework.context.annotation.internalConfigurationAnnotationProcessor org.springframework.context.annotation.internalAutowiredAnnotationProcessor org.springframework.context.annotation.internalCommonAnnotationProcessor org.springframework.context.event.internalEventListenerProcessor org.springframework.context.event.internalEventListenerFactory bean1 bean2 =============添加 bean 后处理器 =================== 20:18:25.368 [main] DEBUG org.springframework.beans.factory.support.DefaultListableBeanFactory - Creating shared instance of singleton bean 'org.springframework.context.annotation.internalAutowiredAnnotationProcessor' 20:18:25.369 [main] DEBUG org.springframework.beans.factory.support.DefaultListableBeanFactory - Creating shared instance of singleton bean 'org.springframework.context.annotation.internalCommonAnnotationProcessor' config org.springframework.context.annotation.internalConfigurationAnnotationProcessor org.springframework.context.annotation.internalAutowiredAnnotationProcessor org.springframework.context.annotation.internalCommonAnnotationProcessor org.springframework.context.event.internalEventListenerProcessor org.springframework.context.event.internalEventListenerFactory bean1 bean2 ================查看输出================ config org.springframework.context.annotation.internalConfigurationAnnotationProcessor org.springframework.context.annotation.internalAutowiredAnnotationProcessor org.springframework.context.annotation.internalCommonAnnotationProcessor org.springframework.context.event.internalEventListenerProcessor org.springframework.context.event.internalEventListenerFactory bean1 bean2 20:18:25.372 [main] DEBUG org.springframework.beans.factory.support.DefaultListableBeanFactory - Creating shared instance of singleton bean 'bean1' 20:18:25.372 [main] DEBUG org.springframework.beans.factory.support.DefaultListableBeanFactory - Creating shared instance of singleton bean 'config' 20:18:25.382 [main] DEBUG com.lou.springboot.Application$Bean1 - 构造 Bean1 20:18:25.387 [main] DEBUG org.springframework.beans.factory.support.DefaultListableBeanFactory - Creating shared instance of singleton bean 'bean2' 20:18:25.388 [main] DEBUG com.lou.springboot.Application$Bean2 - 构造 Bean2 com.lou.springboot.Application$Bean2@351d0846
|
分析
- 初始化
config
- 此时,
beanFactory
注册名字为 config
的 Config
的类
- 看输出此时只有
config
- 添加后处理器
- 添加
beanFactory
后处理器
- 添加 bean 后处理器
- 查看输出
- 这里有一个特别的注意的地方,注册到
beanFactory
后,并没有实例化,只有用到的时候才实例化。「输出构造的语句」
- 如果想要提前实例化可以替换下面的代码
1 2 3 4 5
| System.out.println("================查看输出================");
beanFactory.preInstantiateSingletons();
System.out.println(beanFactory.getBean(Bean1.class).getBean2());
|
BeanFactory
是一个非常低层的类,如果要使用,需要自己走上面的步骤才行,但是,BeanFactory
的实现类 ApplicationContext
帮我们把上面的步骤都做好了,可以直接用。